6. 0110 - Loops
What’s the Point?
-
Understand the purpose of loops
-
Understand the difference between definite and indefinite loops
-
Use
while
loops -
Use
do-while
loops -
Use
for
loops
Source code examples from this chapter and associated videos are available on GitHub.
A computer is designed to execute a series of instructions, in order, very quickly. Now that we understand how to use Boolean expressions to control the flow of our programs, we can use that concept for a programming structure that really unlocks the power of the computer: repetition. Many of the tasks we think of as "computer tasks" are repetitive in nature. Processing data often involves performing the same operation on each piece of information, one after the other, until everything is processed. A computer game has to draw the screen, check for collisions, check what the user is doing with the gamepad, and update the positions of all the objects on the screen, over and over again, until the game ends. When we use a search engine on the web, that search engine has scanned the web one page at a time, indexing what it finds and then moving on to the next page, until it’s scanned all of the pages in its database.
Computers are great for these kinds of tasks: they don’t get bored, they don’t get tired, and they don’t make mistakes—other than the mistakes we make when programming them!
To create the kind of repetition that leverages this processing power, programmers use loops. A loop is a structure that repeats a block of code as long as a certain condition is true. Each time the loop repeats, it’s called an iteration.
6.1. while
Loops
The first structure Java offers for creating loops is the while
loop.
The while
loop repeats a block of code as long as a specified condition is true.
The condition is checked before the block of code is executed, so the block of code might not execute at all if the condition is false the first time it’s checked.
A while
loop is exactly like an if
statement: a Boolean expression is checked, and if it’s true, the block of code is executed.
The only difference is that, after the block of code is executed, the program jumps back to the beginning of the loop and checks the condition again.
If the condition is still true, the block of code is executed again.
while
loop1
2
3
4
5
int count = 0;
while (count < 10) {
System.out.println("Count is: " + count);
count++;
}
In this example, the Boolean expression count < 10
is checked; if it’s true, the block of code is executed.
The block of code prints out the value of count
, then increments count
by 1.
The program then jumps back to the beginning of the loop and checks the condition again.
This process repeats until the expression count < 10
is false.
The result of this loop is that the program prints out the value of count
10 times, starting with 0 and ending with 9.
It is a definite loop because, before the loop starts, it’s already known that the loop will repeat 10 times.
6.2. Infinite Loops
One thing to be careful of when using a while
loop is the possibility of creating an infinite loop.
An infinite loop is a loop that repeats forever, because the condition that determines whether the loop should repeat is never false.
Infinite loops are a common mistake for new programmers, and they can cause our program to hang or crash.
1
2
3
4
int count = 0;
while (count < 10) {
System.out.println("Count is: " + count);
}
In this example, we’ve forgotten to increment count
by 1 after printing it out.
count
will always be 0
, so the condition count < 10
will always be true.
The program will print out "Count is: 0" over and over again, forever.
Your IDE will have a way to stop the program if it’s stuck in an infinite loop. Often, this is a square button—essentially the symbol for "stop". In VS Code, you can also kill the terminal window that’s running the program by clicking the trash can icon in the terminal window. Finally, in many cases you can press Ctrl+C to stop the program. |
The simplest infinite loop we can create in Java is:
1
2
3
while (true) {
// hey, there's nothing to stop this loop!
}
This loop will repeat forever, because the condition true
is always true.
Some programmers use this to start a loop and then use an if statement to break out of the loop when a certain condition is met, but that’s not really an infinite loop—it just puts the boolean expression that controls the loop in an if
statement within the loop’s body instead of in the while
statement.
I personally consider that less readable than using a clear condition in the while
statement, so I don’t write loops like that.
6.3. do-while
Loops
As we’ve seen, a while
loop checks the condition before executing the block of code.
A do-while
loop is similar, but it checks the condition after executing the block of code: run first, then check.
This means that the block of code will always execute at least once.
Other than that, a do-while
loop is exactly the same as a while
loop.
do-while
loop1
2
3
4
5
int count = 0;
do {
System.out.println("Count is: " + count);
count++;
} while (count < 10);
This is the same loop as the while
loop we looked at earlier, but the condition is checked after the block of code is executed.
The while
statement is at the end of the loop; the do
statement at the beginning indicates the block of code that should iterate.
6.3.1. Choosing Between a while
and a do-while
Loops
Both while
and do-while
loops work well for indefinite loops (though they can be used for definite loops as well).
There’s nothing in the structure of these loops that requires a counter or other control variable, so they can be used for loops that repeat until a certain condition is met, however many iterations that requires.
In many cases, it doesn’t matter whether we use a while
or a do-while
loop.
We really can use either one to create the same loop.
However, in some cases, one might be a better choice than the other.
The simple rule of thumb for now is: if we need to guarantee that the block of code will execute at least once, we should use a do-while
loop; if we need to check the condition before executing the block of code, we should use a while
loop.
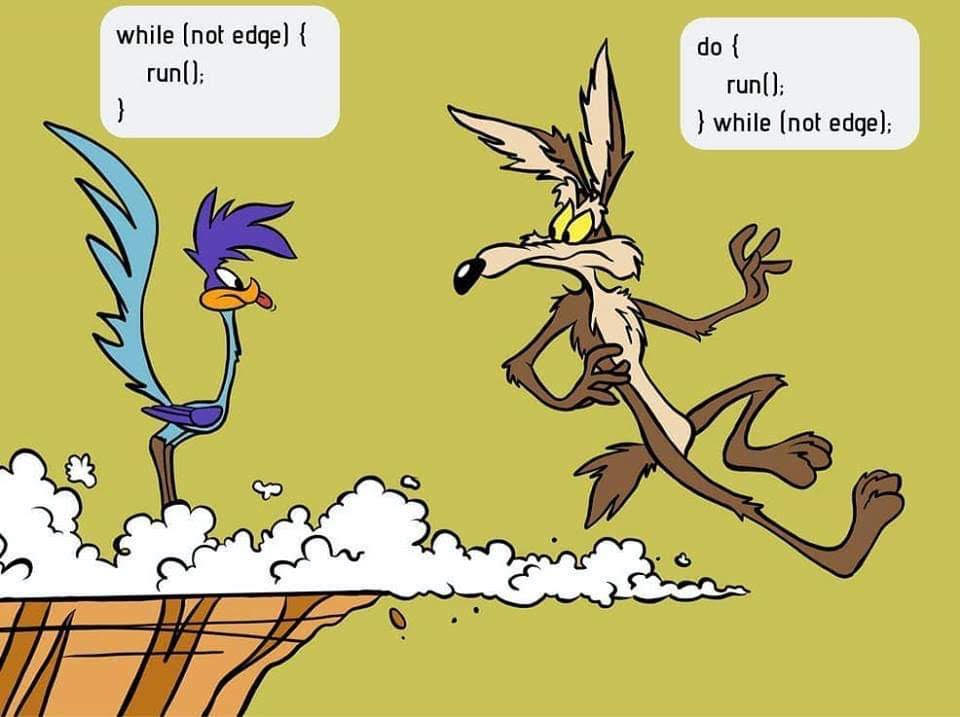
6.4. Input Validation with Loops
A common use for indefinite loops is input validation. Input validation is the process of checking the data that a user inputs into a program to make sure it’s valid. For example, if a program displays a menu with three options, a loop could keep asking for a selection until the user enters one of the three choices.
There are more advanced techniques we’ll eventually use for input validation, but indefinite loops are a simple way to make sure the user’s input is what we expect.
do-while
loop 1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
import java.util.Scanner;
public class InputValidation {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int choice;
do {
System.out.println("Choose an option:");
System.out.println("1. Check balance");
System.out.println("2. Deposit");
System.out.println("3. Withdraw");
System.out.println("4. Exit");
System.out.print("Your choice: ");
choice = input.nextInt();
} while (choice < 1 || choice > 4);
// Rest of the code...
}
}
This snippet of a program will keep displaying the menu and asking for the user’s choice until the user enters a number between 1 and 4.
6.5. for
Loops
Definite loops are really common, especially when we learn about things like arrays later on, so Java provides a keyword that allows for a compact format to create that kind of loop: the for
loop.
The syntax of a for
loop can be a little intimidating for new coders, but it really just combines into one line of code all three of the pieces we need for a loop: initializing a counter, checking the counter, and changing the counter.
for
loop syntax1
2
3
for (int count = 0; count < 10; count++) {
System.out.println("Count is: " + count);
}
The for
loop has three parts, separated by semicolons:
1. Initialize a counter. Example: int count = 0
.
2. A Boolean expression that determines if the loop should repeat. Example: count < 10
.
3. Change the counter at the end of the iteration. Example: count++
.
Once you get the hang of the syntax, the for
loop is a really convenient way to create a definite loop.
6.6. OPTIONAL: break
and continue
Statements
I believe that while
, do-while
, and for
loops, when written with clear Boolean expressions, are the most readable loops, and any loop a coder will need in their career can be written with those structures.
A well-written loop will execute the block of code as many times as necessary, and then stop when the condition is false, without any additional help from the programmer.
However, Java provides two statements that can be used to alter the flow of a loop: the break
statement and the continue
statement.
Since they aren’t necessary for writing loops, I consider them optional: none of my assignments or quiz questions will require you to know them.
The break
statement is used to exit a loop early.
When the break
statement is executed, the loop stops, and the program continues with the next statement after the loop; think of it as a return
statement for a loop (except that it can’t pass a value anywhere).
Some programmers use break
when they need to get out of a loop before the controlling condition is false.
My own opinion is that this is a sign the controlling condition should be rethought, but because you’re likely to see break
in other people’s code, I think it’s important to know about it.
The continue
statement is used to skip the rest of the block of code in a loop and jump back to the beginning of the loop.
When the continue
statement is executed, the program stops executing the loop’s block of code, and jumps to the Boolean expression that controls the loop to see if it should run again.
6.7. A Word About Nested Loops
We can put a loop inside another loop, and that’s called a nested loop. They are useful in some situations, and studying them can improve your ability to think through loop-based algorithms.
However, they are beyond the scope of this course, which focuses on the fundamentals of object-oriented programming.
If you want to explore them, the textbook addresses nested loops in section 6.6 (page 220), and there are many great resources available on the web and YouTube.
6.8. JUST FOR FUN: Recursion
I plan to record a brief video on recursion, but it’s not a high priority. This topic is not covered in the textbook, so if you really can’t wait, search for the topic on the internet.
6.9. Solution Walkthroughs
In "solution walkthrough" videos, I give a problem/prompt that is similar to the kinds of work I assign, and then I record myself writing a solution. It’s not absolutely mandatory to watch this video, but students report that these videos are particularly helpful.
Check Yourself Before You Wreck Yourself (on the assignments)
Can you answer these questions?
Sample answers provided in Stuff That’s Tacked On The End.